函数
函数的定义
1 | def 函数名([输入参数]): |
上述输入参数
表示的是形式参数,简称形参
调用函数的调用者传入的数值,称为实际参数,简称实参。实参的位置是函数的调用处。
函数的参数传递
位置实参
根据形参对应的位置进行实参传递
1 | # 自定义函数 |
关键字实参
根据形参名称进行实参传递
1 | # 自定义函数 |
函数的参数传递——可变实参与不可变实参
1 | # 自定义函数 |
上述代码中,fun() 函数中的形参 arg1 的作用域只在函数体内,fun() 函数中的形参 arg2 是数组的地址,因此操作数组地址对应的数组内容,则影响所有使用到该数组地址的地方。
使用图解描述上述的现象:
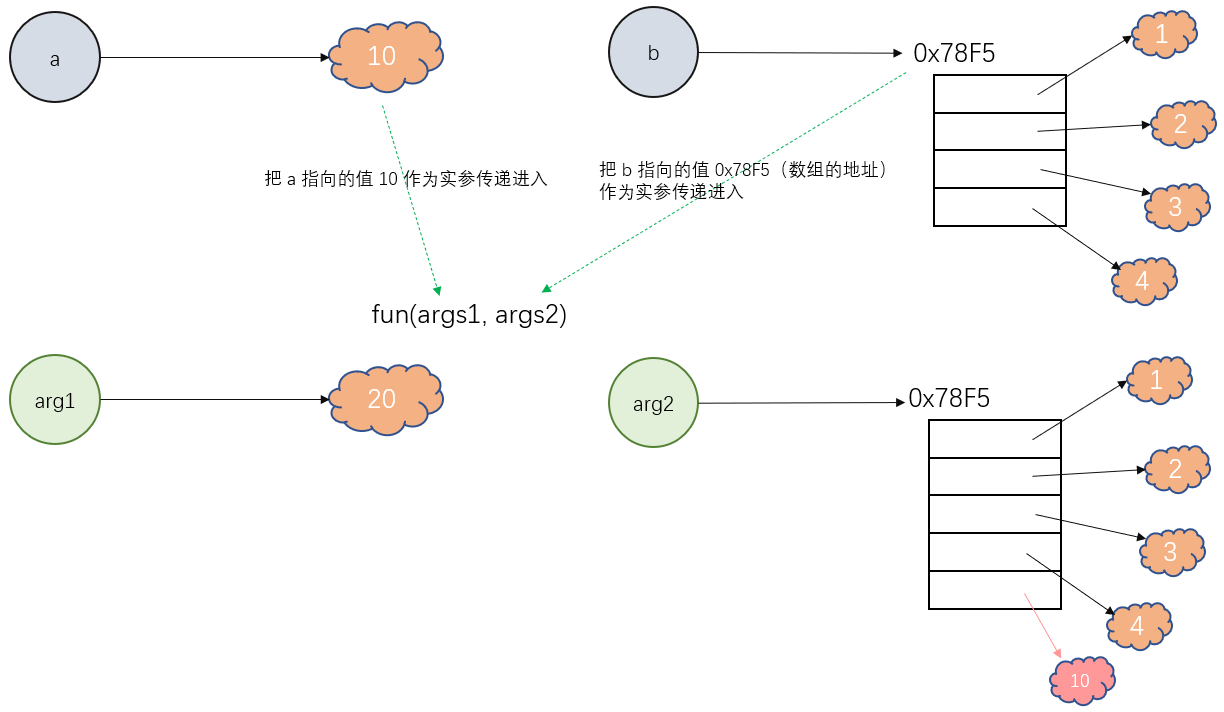
函数的返回值
如果函数没有返回值,return 可以省略不写。如果调用者一定要接受没有返回值的函数,则返回
None
。函数的返回值,如果是 1 个,直接返回类型。
函数的返回值,如果是多个,返回的数据类型为元组。
无返回值,示例:
1 | # 自定义函数 |
只有一个返回值,示例:
1 | # 自定义函数 |
多个返回值,示例:
1 | # 自定义函数 |
函数参数
位置参数
1 | def power(x, n): |
默认参数
1 | def power(x, n=2): |
设置默认参数时,有几点要注意:
必选参数在前,默认参数在后,否则Python的解释器会报错。
如何设置默认参数:当函数有多个参数时,把变化大的参数放前面,变化小的参数放后面。变化小的参数就可以作为默认参数。
个数可变的位置参数
定义函数时,可能无法事先确定传递的位置实参的个数时,使用可变的位置参数
使用
*
定义个数可变的位置形参结果为一个元组
1 | def fun(*args): |
如果想把 list 中每一个元素传入 fun() 函数中,则需要在 list 变量之前加*
1 | def fun(*args): |
个数可变的关键字参数
- 定义函数时,可能无法事先确定传递的关键字实参的个数时,使用可变的关键字参数
- 使用
**
定义个数可变的关键字形参 - 结果为一个字典
1 | def fun(**kw): |
如果想把 dict 中每一个元素传入 fun() 函数中,则需要在 dict 变量之前加**
1 | def fun(**kw): |
命名关键字参数
命名关键字参数需要一个特殊分隔符*
,*
后面的参数被视为命名关键字参数。
1 | def fun(x, y, *, name, age): |
如果函数定义中已经有了一个可变参数,后面跟着的命名关键字参数就不再需要一个特殊分隔符*
了:
1 | def person(name, age, *args, city, job): |
多种参数的组合
在 Python 中定义函数,可以用必选参数、默认参数、可变参数、关键字参数和命名关键字参数,这5种参数都可以组合使用。但是请注意,参数定义的顺序必须是:必选参数、默认参数、可变参数、命名关键字参数和关键字参数。
比如定义一个函数,包含上述若干种参数:
1 | def f1(a, b, c=0, *args, **kw): |
变量的作用域
函数体外的变量
函数体之外的变量可以作用于函数体内外:
1 | name = 'woodwhales.cn' |
函数体内的变量
函数体内的变量只作用于函数体内,称为局部变量。如果想让函数体内的变量能在函数体之外被使用到,则需要给局部变量前面加global
1 | def fun(): |